Intro
This is basically the same post as my previous post, Raspberry Pi photo frame using your pictures on your Google Drive. The are several ideas I am introducing in this treatment.
- better time separation of the photos for a more meaningful viewing
- smart resizing of photos to effectively enlarge narrow photos
- analysis of photos for date and time
- analysis of photos for GPS info, converting to city and even address!
- build up of alternate slideshow which includes date, file, folder and location information embedded at the bottom of every picture
- quality control check to make sure file is an actual JPEG
- tiny thumbnail pictures are skipped
- rotating slideshow refreshes either daily or every three days
- pictures of documents are excluded (future enhancement)
- a nice picture is displayed when the slideshow is refreshed
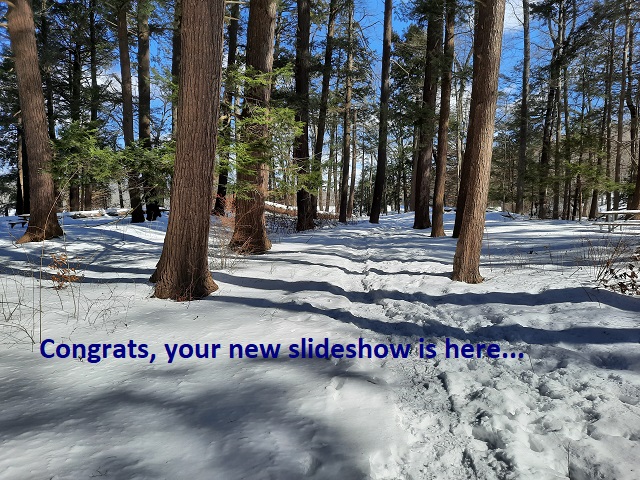
Mostly for my own sake, I’ve re-named most of the relevant files and re-worked some as well in order to avoid name conflicts.
I find this treatment is pretty robust and can withstand a lot of errors and mistakes.
So let’s get started.
The easier way to get the files
Because there are now so many files – 18 at last count! – I’ve bundled them all into a tar file. So to get them all in one fell swoop do this.
$ wget https://drjohnstechtalk.com/blog/downloads/photoFrameII.tar
$ tar xvf photoFrameII.tar
Then skip down to the section of this post called crontab entries, which you will still need to do.
But because I think the scripts could be useful for other projects as well, I’m including them here in their entirety in the following section.
The files
The brains of the thing is master3.sh.
master3.sh
#!/bin/sh
# DrJ 1/2021
# call this from cron once a day to refesh random slideshow once a day
NUMFOLDERS=20
DEBUG=1
HOME=/home/pi
RANFILE=$HOME/random.list
REANFILE=$HOME/rean.list
DISPLAYFOLDER=$HOME/Pictures
DISPLAYFOLDERTMP=$HOME/Picturestmp
EXIFTMP=$HOME/EXIFtmp
EXIF=$HOME/EXIF
TXTDIR=$HOME/picstxt
MSHOW=$HOME/mediashow
MSHOW2=$HOME/mediashowtmp2
MSHOW3=$HOME/mediashowtmp3
SLEEPINTERVAL=1
STARTFOLDER="MaryDocs/Pictures and videos"
echo "Starting master process at "`date`
cd $HOME
rm -rf $DISPLAYFOLDERTMP
mkdir $DISPLAYFOLDERTMP
#listing of all Google drive files starting from the picture root
# this takes a few minutes so we may want to skip for debugging
if [ "$1" = "skip" ]; then
if [ $DEBUG -eq 1 ]; then echo SKIP Listing all files from Google drive; fi
else
if [ $DEBUG -eq 1 ]; then echo Listing all files from Google drive; fi
rclone ls remote:"$STARTFOLDER" > files
# filter down to only jpegs, lose the docs folders and the tiny JPEGs
if [ $DEBUG -eq 1 ]; then echo Picking out the JPEGs and losing the small images; fi
egrep '\.[jJ][pP][eE]?[gG]$' files |awk '$1 > 11000 {$1=""; print substr($0,2)}'|grep -i -v /docs/ > jpegs.list
fi
# check if we got anything. If our Internt dropped there may have been a problem, for instance
flines=`cat files|wc -l`
if [ $flines -lt 60 ]; then
echo "rclone did not produce enough files. Check your Internet setup and rclone configuration."
echo Only $flines files in the file listing - not enough - so pausing 60 seconds and starting over... at `date`
# start a new job and kill ourselves!
nohup $HOME/master3.sh > master.log 2>&1 &
exit
fi
# throw NUMFOLDERS or so random numbers for picture selection, select triplets of photos by putting
# names into a file
if [ $DEBUG -eq 1 ]; then echo "\nGenerate random filename triplets"; fi
./random-files3.pl -f $NUMFOLDERS -j jpegs.list -r $RANFILE
# copy over these 60 jpegs
if [ $DEBUG -eq 1 ]; then echo "\nCopy over these random files"; fi
cat $RANFILE|while read line; do
if [ $DEBUG -eq 1 ]; then echo filepath is $line; fi
rclone copy remote:"${STARTFOLDER}/$line" $DISPLAYFOLDERTMP
sleep $SLEEPINTERVAL
done
# do a re-analysis to push pictures further apart in time
if [ $DEBUG -eq 1 ]; then echo "\nRe-analyzing pictures for their timestamps"; fi
cd $DISPLAYFOLDERTMP; $HOME/reanalyze.pl
# copy over just the new pictures that we determined were needed
if [ $DEBUG -eq 1 ]; then echo "\nCopy over the needed replacement files"; fi
cat $REANFILE|while read line; do
if [ $DEBUG -eq 1 ]; then echo filepath is $line; fi
rclone copy remote:"${STARTFOLDER}/$line" $DISPLAYFOLDERTMP
sleep $SLEEPINTERVAL
done
# QC: toss out the pics which are not actually JPEGs
if [ $DEBUG -eq 1 ]; then echo "\nQC: Toss out the pics which are not actually JPEGs"; fi
cd $DISPLAYFOLDERTMP; ../QC.pl
# save EXIF metadata for later
if [ $DEBUG -eq 1 ]; then echo "\nSave EXIF metadata for later"; fi
cd $DISPLAYFOLDERTMP; $HOME/get-all-EXIF.sh
rm -rf $EXIF;mv $EXIFTMP $EXIF
# analyze EXIF info to extract most interesting things
if [ $DEBUG -eq 1 ]; then echo "\nAnalyze EXIF data"; fi
rm -rf $TXTDIR; $HOME/analyze.sh
# rotate pics as needed
if [ $DEBUG -eq 1 ]; then echo "\nRotate the pics which need it"; fi
cd $DISPLAYFOLDERTMP; $HOME/rotate-as-needed.sh
# resize pics
if [ $DEBUG -eq 1 ]; then echo "\nSize all pics to the display size"; fi
$HOME/resize.sh
# create text info + images
if [ $DEBUG -eq 1 ]; then echo "\nEmbed pic info"; fi
$HOME/embedpicinfo.sh
cd ~
# kill any old slideshow
if [ $DEBUG -eq 1 ]; then echo Killing old fbi slideshow; fi
sudo pkill -9 -f fbi
pkill -9 -f m3.pl
# remove old pics
if [ $DEBUG -eq 1 ]; then echo Removing old pictures; fi
rm -rf $DISPLAYFOLDER
mv $DISPLAYFOLDERTMP $DISPLAYFOLDER
cp $MSHOW3 $MSHOW
touch refresh
#run looping fbi slideshow on these pictures
if [ $DEBUG -eq 1 ]; then echo Start "\nfbi slideshow in background"; fi
cd $DISPLAYFOLDER ; nohup ~/m3.pl >> ~/m3.log 2>&1 &
if [ $DEBUG -eq 1 ]; then echo "And now it is "`date`; fi
random-files3.pl
#!/usr/bin/perl
use Getopt::Std;
my %opt=();
getopts("c:df:j:r:",\%opt);
$nofolders = $opt{f} ? $opt{f} : 20;
$DEBUG = $opt{d} ? 1 : 0;
$cutoff = $opt{c} ? $opt{c} : 5;
$cutoffS = 60*$cutoff;
$jpegs = $opt{j} ? $opt{j} : "jpegs.list";
$ranpicfile = $opt{r} ? $opt{r} : "jpegs-random.list";
print "d,f,j,r: $opt{d}, $opt{f}, $opt{j}, $opt{r}\n" if $DEBUG;
$mshowt = "mediashowtmp";
open(JPEGS,$jpegs) || die "Cannot open jpegs listing file $jpegs!!\n";
@jpegs = <JPEGS>;
# remove newline character
$nopics = chomp @jpegs;
open(RAN,"> $ranpicfile") || die "Cannot open random picture file $ranpicfile!!\n";
for($i=0;$i<$nofolders;$i++) {
$t = int(rand($nopics-2));
print "random number is: $t\n" if $DEBUG;
# a lot of our pics follow this naming convention
# 20160831_090658.jpg
($date,$time) = $jpegs[$t] =~ /(\d{8})_(\d{6})/;
if ($date) {
print "date, time: $date $time\n" if $DEBUG;
# ensure neighboring picture is at least five minutes different in time
$iPO = $iP = $diff = 1;
($hr,$min,$sec) = $time =~ /(\d\d)(\d\d)(\d\d)/;
$secs = 3600*$hr + 60*$min + $sec;
print "Pre-pic logic\n";
while ($diff < $cutoffS) {
$iP++;
$priorPic = $jpegs[$t-$iP];
$Pdate = $Ptime = 0;
($Pdate,$Ptime) = $priorPic =~ /(\d{8})_(\d{6})/;
($Phr,$Pmin,$Psec) = $Ptime =~ /(\d\d)(\d\d)(\d\d)/;
$Psecs = 3600*$Phr + 60*$Pmin + $Psec;
print "hr,min,sec,Phr,Pmin,Psec: $hr,$min,$sec,$Phr,$Pmin,$Psec\n" if $DEBUG;
$diff = abs($secs - $Psecs);
print "diff: $diff\n" if $DEBUG;
# end our search if we happened upon different dates
$diff = 99999 if $Pdate ne $date;
}
# post-picture logic - same as pre-picture
print "Post-pic logic\n";
$diff = 0;
while ($diff < $cutoffS) {
$iPO++;
$postPic = $jpegs[$t+$iPO];
$Pdate = $Ptime = 0;
($Pdate,$Ptime) = $postPic =~ /(\d{8})_(\d{6})/;
($Phr,$Pmin,$Psec) = $Ptime =~ /(\d\d)(\d\d)(\d\d)/;
$Psecs = 3600*$Phr + 60*$Pmin + $Psec;
print "hr,min,sec,Phr,Pmin,Psec: $hr,$min,$sec,$Phr,$Pmin,$Psec\n" if $DEBUG;
$diff = abs($Psecs - $secs);
print "diff: $diff\n" if $DEBUG;
# end our search if we happened upon different dates
$diff = 99999 if $Pdate ne $date;
}
} else {
$iP = $iPO = 2;
}
$priorPic = $jpegs[$t-$iP];
$Pic = $jpegs[$t];
$postPic = $jpegs[$t+$iPO];
print RAN qq($priorPic
$Pic
$postPic
);
# this is how we'll preserve the order of the pictures. ls -1 often gives a different order!!
($p1) = $priorPic =~ /([^\/]+)$/;
($p2) = $Pic =~ /([^\/]+)$/;
($p3) = $postPic =~ /([^\/]+)$/;
print "p1 p2 p3: $p1 $p2 $p3" if $DEBUG;
$picsinorder .= $p1 . "\0" . $p2 . "\0" . $p3 . "\0";
}
close(RAN);
open(MS,">$mshowt") || die "Cannot open mediashow file $mshowt!!\n";
print MS $picsinorder;
close(MS);
print "pics in order: $picsinorder\n" if $DEBUG;
reanalyze.pl
#!/usr/bin/perl
use Getopt::Std;
my %opt=();
#
# assumption is that we are runnin this from a directory containing pictures
$tier1 = 100; $tier2 = 200; $tier3 = 300; # secs
$DEBUG = 1;
$HOME = "/home/pi";
# pics are here
$pNames = "$HOME/reanpicnames";
$ranfile = "$HOME/random.list";
$reanfile = "$HOME/rean.list";
$origfile = "$HOME/jpegs.list";
$mshowt = "$HOME/mediashowtmp";
$mshow2 = "$HOME/mediashowtmp2";
open(REAN,">$reanfile") || die "Cannot open reanalyze file $reanfile!!\n";
$ms = `cat $mshowt`;
print "Original media show: $ms\n" if $DEBUG;
@lines = split('\0',$ms);
$Pdate = $Phr = $Pmin = $Psec = 0;
$diff = 9999;
for($i=0;$i<@lines;$i++){
$date = 0;
$secs = $ymd = 0;
$_ = $lines[$i];
$file = $_;
# ignore pictures with names like 20130820_180050.jpg
next if /\d{8}_\d{4}/;
open(ANAL,"$HOME/getinfo.py \"$file\"|") || die "Cannot open file: $file!!\n";
print "filename: $file\n" if $DEBUG;
while(<ANAL>){
#extract date and time from remaining pictures, if possible
# # DateTimeOriginal = 2018:08:18 20:16:47
# print STDERR "DATE: $_" if $DEBUG;
if (/date/i && $date++ < 1) {
print "date match in getinfo.pyoutput: $_" if $DEBUG;
($ymd,$hr,$min,$sec) = /(\d{4}:\d\d:\d\d) (\d\d):(\d\d):(\d\d)/;
$secs = 3600*$hr + 60*$min + $sec;
print "file,secs,ymd,i: $file,$secs,$ymd,$i\n" if $DEBUG;
$YMD[$i] = $ymd;
$SECS[$i] = $secs;
}
} # end loop over analysis of this pic
} # end loop over all files
# now go over that
$oldfolder = 0;
for($i=1;$i<@lines;$i++){
$folder = int($i/3) + 1;
next unless $folder != $oldfolder;
print "analyzing results. folder no. $folder\n" if $DEBUG;
# analyze pics in triplets
# center pic
$j = ($folder - 1)*3 + 1;
for ($o=-1;$o<2;$o+=2){
$k=$j+$o;
print "j,k,o: $j,$k,$o\n" if $DEBUG;
next unless $SECS[$j] > 0 && $YMD[$j] == $YMD[$k] && $YMD[$j] > 0;
print "We have non-0 dates we're dealing with\n" if $DEBUG;
$file = $lines[$k];
chomp($file);
$diff = abs($SECS[$j] - $SECS[$k]);
print "diff: $diff\n" if $DEBUG;
next unless $diff < $tier3;
# the closer the files are together the more we push away
$bump = 1 if $diff < $tier3;
$bump = 2 if $diff < $tier2;
$bump = 3 if $diff < $tier1;
# get full filepath
$filepath = `grep \"$file\" $ranfile`;
chomp($filepath);
# now use that to search within the jpegs file listing
$prog = $o < 0 ? "head" : "tail";
$newfilepath = `grep -C$bump "$filepath" $origfile|$prog -1`;
($newfile) = $newfilepath =~ /([^\/]+)$/;
chomp($newfile);
print "file,filepath,newfile,newfilepath,bump: $file,$filepath,$newfile,$newfilepath,$bump\n" if $DEBUG;
print REAN $newfilepath;
# we'll get the new pictures over in a separate step to keep this more atomic
$ms =~ s/$file/$newfile/;
}
$oldfolder = $folder;
} # end loop over pics
# print out new mediashow pics in order
print "Printing new mediashow: $ms\n" if $DEBUG;
open(MS,">$mshow2") || die "Cannot open mediashow $mshow2!!\n";
print MS $ms;
close(MS)
QC.pl
#!/usr/bin/perl
# kick out the non-JPEG files - sometimes they creep in
$DEBUG = 1;
$HOME = "/home/pi";
$mshow2 = "$HOME/mediashowtmp2";
$mshow3 = "$HOME/mediashowtmp3";
$ms = `cat $mshow2`;
@pics = split('\0',$ms);
foreach $file (@pics) {
print "file is $file\n" if $DEBUG;
#DSC00185.JPG: JPEG image data, JFIF standard 1.01...
$res = `file "$file"|cut -d: -f2`;
if ($res =~ /JPEG/i){
print "This file is indeed a JPEG image\n" if $DEBUG;
} else {
print "Not a JPEG image! We have to remove this file form the mediashow\n" if $DEBUG;
$ms =~ s/$file\0//;
}
}
# print out new mediashow pics in order
print "Printing new mediashow: $ms\n" if $DEBUG;
open(MS,">$mshow3") || die "Cannot open mediashow $mshow3!!\n";
print MS $ms;
close(MS);
get-all-EXIF.sh
#!/bin/sh
# DrJ 1/2021
# preserve EXIF info of all the images because our rotate step removes it
# and we will use it in subsequent steps
# assumption is that our current directory is the one where we want to read files
EXIFTMP=~/EXIFtmp
mkdir $EXIFTMP
ls -1|while read line; do
echo file is "$line"
~/getinfo.py "$line" > $EXIFTMP/"$line"
done
analyze.sh
#!/bin/sh
# DrJ 1/2021
# try to extract date, file and folder name and even GPS info, create jpegs with info
# for each image
# assumption is that are current directory is the one where we want to alter files
HOME=/home/pi
TXTDIR=$HOME/picstxt
# it's assumed EXIF info for each pic has already been extracted and put into EXIF diretory
EXIF=$HOME/EXIF
mkdir $TXTDIR
cd $EXIF
ls -1|while read line; do
echo file is "$line"
echo -n "$line"|../analyzeDate.pl > "$TXTDIR/${line}"
echo -n "$line"|../analyzeGPS.pl >> "$TXTDIR/${line}"
done
rotate-as-needed.sh
#!/bin/sh
# DrJ 12/2020
# some of our downloaded files will be sideways, and fbi doesn't auto-rotate them as far as I know
# assumption is that our current directory is the one where we want to alter files
ls -1|while read line; do
echo file is "$line"
o=`~/getinfo.py "$line"|grep -ai orientation|awk '{print $NF}'`
echo orientation is $o
if [ "$o" -eq "6" ]; then
echo "90 clockwise is needed, o is $o"
# rotate and move it
~/rotate.py -90 "$line"
mv rot_"$line" "$line"
elif [ "$o" -eq "8" ]; then
echo "90 counterclock is needed, o is $o"
# rotate and move it
~/rotate.py 90 "$line"
mv rot_"$line" "$line"
elif [ "$o" -eq "3" ]; then
echo "180 rot is needed, o is $o"
# rotate and move it
~/rotate.py 180 "$line"
mv rot_"$line" "$line"
fi
done
resize.sh
#!/bin/sh
# DrJ 2/2021
# To combat the RPi's inherent sluggish performance we'll downsize the pictures in advance to save fbi the effort
#
# on the pidisplay fbset gives:
#mode "800x480"
# geometry 800 480 800 480 32
# timings 0 0 0 0 0 0 0
# rgba 8/16,8/8,8/0,8/24
#endmode
displaywidth=`fbset|grep geometry|awk '{print $2}'`
displayheight=`fbset|grep geometry|awk '{print $3}'`
ls -1|while read line; do
echo file is "$line"
~/fancyresize.py $displaywidth $displayheight "$line"
mv resize_"$line" "$line"
done
embedpicinfo.sh
#!/bin/sh
# DrJ 2/2021
# To combat the RPi's inherent sluggish performance we'll downsize the pictures in advance to save fbi the effort
#
# on the pidisplay fbset gives:
#mode "800x480"
# geometry 800 480 800 480 32
# timings 0 0 0 0 0 0 0
# rgba 8/16,8/8,8/0,8/24
#endmode
displaywidth=`fbset|grep geometry|awk '{print $2}'`
displayheight=`fbset|grep geometry|awk '{print $3}'`
ls -1|while read line; do
echo file is "$line"
# this will create a new image with same name prepended with txt_
~/embedpicinfo.py $displaywidth $displayheight "$line"
done
Auxiliary files
rotate.py
#!/usr/bin/python3
# call with two arguments: degrees-to-rotate and filename
import PIL, os
import sys
from PIL import Image
# first do: pip3 install piexif
import piexif
degrees = int(sys.argv[1])
pic = sys.argv[2]
picture= Image.open(pic)
# see https://github.com/hMatoba/Piexif for piexif writeup
# this method of preserving EXIF info does not always work, and
# causes script to crash when it fails!
##exif_dict = piexif.load(picture.info["exif"])
##exif_bytes = piexif.dump(exif_dict)
## both rotate and preserve EXIF data
##picture.rotate(degrees,expand=True).save("rot_" + pic,"jpeg", exif=exif_bytes)
# rotate (which will blow away EXIF info, sorry...)
picture.rotate(degrees,expand=True).save("rot_" + pic,"jpeg")
getinfo.py
#!/usr/bin/python3
import os,sys
from PIL import Image
from PIL.ExifTags import TAGS
for (tag,value) in Image.open(sys.argv[1])._getexif().items():
print ('%s = %s' % (TAGS.get(tag), value))
print ('%s = %s' % (TAGS.get(tag), value))
embedpicinfo.py
#!/usr/bin/python3
# from https://auth0.com/blog/image-processing-in-python-with-pillow/
# fonts are described here:
# https://pillow.readthedocs.io/en/stable/reference/ImageDraw.html
from PIL import Image, ImageDraw, ImageFont
import sys, os
width = int(sys.argv[1])
height = int(sys.argv[2])
# for Pidisplay:
#width = 800
#height = 480
imageFile = sys.argv[3]
imageandtext = 'txt_' + imageFile
tfile = '../picstxt/' + imageFile
f = open(tfile)
txtlines = f.readlines()
f.close()
# our fonts
fnt = ImageFont.truetype("/usr/share/fonts/truetype/dejavu/DejaVuSans-Bold.ttf", 14)
#fnt = ImageFont.truetype("/usr/share/fonts/truetype/dejavu/DejaVuSans.ttf", 40)
fnt36 = ImageFont.truetype("/usr/share/fonts/truetype/dejavu/DejaVuSans-Bold.ttf", 36)
fnt2 = ImageFont.truetype("/usr/share/fonts/truetype/dejavu/DejaVuSans.ttf", 18)
fntBold = ImageFont.truetype("/usr/share/fonts/truetype/dejavu/DejaVuSans-Bold.ttf", 40)
# PiDisplay resolution is 800x480
margin = 35
# semi-parameterized variables
x0 = margin - 30
x1 = margin -15
cwidth = 6
yline = 27
ychevron=(yline-28)/2
ycoff = 5
yoffset = 12
yrec= -10
rpad = 15
#
textimagey = 130
textimagex = width
# menu items
textimage = Image.new('RGB', (textimagex, textimagey), 'white')
for t in txtlines:
img_draw = ImageDraw.Draw(textimage)
img_draw.text((margin, yoffset), t, font=fnt, fill='MidnightBlue')
yoffset += yline
# merge three images together...
# black background covering whole display:
masterimage = Image.new('RGB',(width,height),'black')
# original image:
oimage = Image.open(imageFile)
# original image width
owidth = oimage.size[0]
# get offset so narrow pictures are centered
xoffset = int((width - owidth)/2)
masterimage.paste(oimage,(xoffset,0))
masterimage.paste(textimage,(0,height - textimagey))
masterimage.save(imageandtext)
fancyresize.py
#!/usr/bin/python3
# DrJ 2/2021
import PIL, os
import sys
from PIL import Image
# somewhat inspired by http://www.riisen.dk/dop/pil.html
# arguments:
# <width> <height> file
# with and height should be provided as values in pixels
# image file should be provided as argument.
# A pidisplay is 800x480
displaywidth = int(sys.argv[1])
displayheight = int(sys.argv[2])
smallscreen = 801
imageFile = sys.argv[3]
im1 = Image.open(imageFile)
narrowmax = .76
blowupfactor = 1.1
# take less from the top than the bottom
topshare = .3
bottomshare = 1.0 - topshare
# for DrJ debugging
DEBUG = True
if DEBUG:
print("display width and height: ",displaywidth,displayheight)
def imgResize(im):
width = im.size[0]
height = im.size[1]
if DEBUG:
print("image width and height: ",width,height)
# If the aspect ratio is wider than the display screen's aspect ratio,
# constrain the width to the display's full width
if width/float(height) > float(displaywidth)/float(displayheight):
if DEBUG:
print("In section width contrained to full width code section")
widthn = displaywidth
heightn = int(height*float(displaywidth)/width)
im5 = im.resize((widthn, heightn), Image.ANTIALIAS) # best down-sizing filter
else:
heightn = displayheight
widthn = int(width*float(displayheight)/height)
if width/float(height) < narrowmax and displaywidth < smallscreen:
# if width is narrow we're losing too much by using the whole picture.
# Blow it up by blowupfactor% if display is small, and crop most of it from the bottom
heightn = int(displayheight*blowupfactor)
widthn = int(width*float(heightn)/height)
im4 = im.resize((widthn, heightn), Image.ANTIALIAS) # best down-sizing filter
top = int(displayheight*(blowupfactor - 1)*topshare)
bottom = int(heightn - displayheight*(blowupfactor - 1)*bottomshare)
if DEBUG:
print("heightn,top,widthn,bottom: ",heightn,top,widthn,bottom)
im5 = im4.crop((0,top,widthn,bottom))
else:
im5 = im.resize((widthn, heightn), Image.ANTIALIAS) # best down-sizing filter
im5.save("resize_" + imageFile)
imgResize(im1)
analyzeDate.pl
#!/usr/bin/perl
# 20180818_201647.jpg
use POSIX;
$DEBUG = 1;
$HOME = "/home/pi";
$random = "$HOME/random.list";
$rean = "$HOME/rean.list";
#$file = "Picturestmp/20180422_134220.jpg";
while(<>){
$GPS = $date = 0;
$gpsinfo = "";
$file = $_;
#open(ANAL,"$HOME/getinfo.py \"$file\"|") || die "Cannot open file: $file!!\n";
open(ANAL,"cat \"$file\"|") || die "Cannot open file: $file!!\n";
print STDERR "filename: $file\n" if $DEBUG;
while(<ANAL>){
$town = "";
if (/DateTimeOriginal/i && $date++ < 1) {
# DateTimeOriginal = 2018:08:18 20:16:47
#or... DateTimeDigitized = 2016/03/07 00:57:49
print STDERR "DATE: $_" if $DEBUG;
($yr,$mon,$date,$hr,$min) = /(\d{4}):(\d\d):(\d\d) (\d\d):(\d\d)/;
print STDERR "$yr,$mon,$date,$hr,$min\n" if $DEBUG;
# my custom format: Saturday, August 18, 2018 8:16 pm
$dateinfo = strftime("%A, %B %d, %Y %l:%M %p", 0, $min, $hr, $date , $mon - 1, $yr - 1900, -1, -1, -1);
}
}
# folder info from random.list
$match = `cat $random $rean|grep "$file"`;
($folder) = $match =~ /(.+)\/[^\/]+/;
print STDERR "matched line, folder: $match, $folder\n" if $DEBUG;
# if no date, use filesystem date
if ( ! $dateinfo ) {
$jpegfile = "../Picturestmp/$file";
$mtime = (stat($jpegfile))[9];
$handtst = `ls -l "$jpegfile"`;
@ltime = localtime $mtime;
$dateinfo = strftime("(guess) %A, %B %d, %Y %l:%M %p",@ltime);
print STDERR "No date info. Use filesystem date. mtime is $mtime. dateinfo: $dateinfo\n";
print STDERR "Hand test of file age: $handtst\n";
}
$dateinfo = $dateinfo || "No date found";
$gpsinfo = $gpsinfo || "No info found";
print qq(File: $file
Folder: $folder
Date: $dateinfo
);
}
analyzeGPS.pl
#!/usr/bin/perl
# use in combination with this post https://drjohnstechtalk.com/blog/2020/12/convert-gps-coordinates-into-town-name/
use POSIX;
$DEBUG = 1;
$HOME = "/home/pi";
#$file = "Pictures/20180422_134220.jpg";
while(<>){
$GPS = $date = 0;
$gpsinfo = "";
$file = $_;
#open(ANAL,"$HOME/getinfo.py \"$file\"|") || die "Cannot open file: $file!!\n";
open(ANAL,"cat \"$file\"|") || die "Cannot open file: $file!!\n";
print STDERR "filename: $file\n" if $DEBUG;
while(<ANAL>){
$postalcode = $town = $name = "";
if (/GPS/i) {
print STDERR "GPS: $_" if $DEBUG;
# GPSInfo = {1: 'N', 2: (39.0, 21.0, 22.5226), 3: 'W', 4: (74.0, 25.0, 40.0267), 5: 1.7, 6: 0.0, 7: (23.0, 4.0, 14.0), 29: '2016:07:22'}
($pole,$deg,$min,$sec,$hemi,$lngdeg,$lngmin,$lngsec) = /1: '([NS])', 2: \(([\d\.]+), ([\d\.]+), ([\d\.]+)...3: '([EW])', 4: \(([\d\.]+), ([\d\.]+), ([\d\.]+)\)/i;
print STDERR "$pole,$deg,$min,$sec,$hemi,$lngdeg,$lngmin,$lngsec\n" if $DEBUG;
$lat = $deg + $min/60.0 + $sec/3600.0;
$lat = -$lat if $pole eq "S";
$lng = $lngdeg + $lngmin/60.0 + $lngsec/3600.0;
$lng = -$lng if $hemi = "W" || $hemi eq "w";
print STDERR "lat,lng: $lat, $lng\n" if $DEBUG;
#$placename = `curl -s "$url"|grep -i toponym`;
next if $lat == 0 && $lng == 0;
# the address API is the most precise
$url = "http://api.geonames.org/address?lat=$lat\&lng=$lng\&username=drjohns";
print STDERR "Url: $url\n" if $DEBUG;
$results = `curl -s "$url"|egrep -i 'street|house|locality|postal|adminName'`;
print STDERR "results: $results\n" if $DEBUG;
($street) = $results =~ /street>(.+)</;
($houseNumber) = $results =~ /houseNumber>(.+)</;
($postalcode) = $results =~ /postalcode>(.+)</;
($state) = $results =~ /adminName1>(.+)</;
($town) = $results =~ /locality>(.+)</;
print STDERR "street, houseNumber, postalcode, state, town: $street, $houseNumber, $postalcode, $state, $town\n" if $DEBUG;
# I think locality is pretty good name. If it exists, don't go further
$postalcode = "" if $town;
if (!$postalcode && !$town){
# we are here if we didn't get interesting results from address reverse loookup, which often happens.
$url = "http://api.geonames.org/extendedFindNearby?lat=$lat\&lng=$lng\&username=drjohns";
print STDERR "Address didn't work out. Trying extendedFindNearby instead. Url: $url\n" if $DEBUG;
$results = `curl -s "$url"`;
# parse results - there may be several objects returned
$topelemnt = $results =~ /<geoname>/i ? "geoname" : "geonames";
@elmnts = ("street","streetnumber","lat","lng","locality","postalcode","countrycode","countryname","name","adminName2","adminName1");
$cnt = xml1levelparse($results,$topelemnt,@elmnts);
@lati = @{ $xmlhash{lat}};
@long = @{ $xmlhash{lng}};
# find the closest entry
$distmax = 1E7;
for($i=0;$i<$cnt;$i++){
$dist = ($lat - $lati[$i])**2 + ($lng - $long[$i])**2;
print STDERR "dist,lati,long: $dist, $lati[$i], $long[$i]\n" if $DEBUG;
if ($dist < $distmax) {
print STDERR "dist < distmax condition. i is: $i\n";
$isave = $i;
}
}
$street = @{ $xmlhash{street}}[$isave];
$houseNumber = @{ $xmlhash{streetnumber}}[$isave];
$admn2 = @{ $xmlhash{adminName2}}[$isave];
$postalcode = @{ $xmlhash{postalcode}}[$isave];
$name = @{ $xmlhash{name}}[$isave];
$countrycode = @{ $xmlhash{countrycode}}[$isave];
$countryname = @{ $xmlhash{countryname}}[$isave];
$state = @{ $xmlhash{adminName1}}[$isave];
print STDERR "street, houseNumber, postalcode, state, admn2, name: $street, $houseNumber, $postalcode, $state, $admn2, $name\n" if $DEBUG;
if ($countrycode ne "US"){
$state .= " $countryname";
}
$state .= " (approximate)";
}
# turn zipcode into town name with this call
if ($postalcode) {
print STDERR "postalcode $postalcode exists, let's convert to a town name\n";
print STDERR "url: $url\n";
$url = "http://api.geonames.org/postalCodeSearch?country=US\&postalcode=$postalcode\&username=drjohns";
$results = `curl -s "$url"|egrep -i 'name|locality|adminName'`;
($town) = $results =~ /<name>(.+)</i;
print STDERR "results,town: $results,$town\n";
}
if (!$town) {
# no town name, use adminname2 which is who knows what in general
print STDERR "Stil no town name. Use adminName2 as next best thing\n";
$town = $admn2;
}
if (!$town) {
# we could be in the ocean! I saw that once, and name was North Atlantic Ocean
print STDERR "Still no town. Try to use name: $name as last resort\n";
$town = $name;
}
$gpsinfo = "$houseNumber $street $town, $state" if $locality || $town;
} # end of GPS info exists condition
} # end loop over ANAL file
$gpsinfo = $gpsinfo || "No info found";
print qq(Location: $gpsinfo
);
} # end loop over STDIN
#####################
# function to parse some xml and fill a hash of arrays
sub xml1levelparse{
# build an array of hashes
$string = shift;
# strip out newline chars
$string =~ s/\n//g;
$parentelement = shift;
@elements = @_;
$i=0;
while($string =~ /<$parentelement>/i){
$i++;
($childelements) = $string =~ /<$parentelement>(.+?)<\/$parentelement>/i;
print STDERR "childelements: $childelements" if $DEBUG;
$string =~ s/<$parentelement>(.+?)<\/$parentelement>//i;
print STDERR "string: $string\n" if $DEBUG;
foreach $element (@elements){
print STDERR "element: $element\n" if $DEBUG;
($value) = $childelements =~ /<$element>([^<]+)<\/$element>/i;
print STDERR "value: $value\n" if $DEBUG;
push @{ $xmlhash{$element} }, $value;
}
} # end of loop over parent elements
return $i;
} # end sub xml1levelparse
m3.pl
#!/usr/bin/perl
# show the pics ; rotate the screen as needed
# for now, assume the display is in a neutral
# orientation at the start
use Time::HiRes qw(usleep);
$DEBUG = 1;
$delay = 6; # seconds between pics
###$delay = 4; # for testing
$mdelay = 200; # milliseconds
$mshow = "$ENV{HOME}/mediashow";
$pNames = "$ENV{HOME}/pNames";
# pics are here
$picsDir = "$ENV{HOME}/Pictures";
$refreshFile = "$ENV{HOME}/refresh";
chdir($picsDir);
$cn = `ls -1|wc -l`;
chomp($cn);
print "$cn files\n" if $DEBUG;
# throw up a first picture - all black. Trick to make black bckgrd permanent
system("sudo fbi -a --noverbose -T 1 $ENV{HOME}/black.jpg");
# see if this is a new batch of pictures
$refresh = (stat($refreshFile))[9];
$now = time();
$diff = $now - $refresh;
print "refresh,now,diff: $refresh, $now, $diff\n" if $DEBUG;
if ($diff < 100){
system("sudo fbi -a --noverbose -T 1 $ENV{HOME}/newslideshowintro.jpg");
sleep(25);
}
system("sudo fbi -a --noverbose -T 1 $ENV{HOME}/black.jpg");
system("sleep 1; sudo killall fbi");
# start infinitely looping fbi slideshow
for (;;) {
# then start slide show
# shell echo cannot work with null character so we need to use a file to store it
system("sudo xargs -a $mshow -0 fbi --noverbose -1 -T 1 -t $delay ");
###system("sudo xargs -a $mshow -0 fbi -a -1 -T 1 -t $delay "); # for testing
# fbi runs in background, then exits, so we need to monitor if it's still alive
for(;;) {
open(MON,"ps -ef|grep fbi|grep -v grep|") || die "Cannot launch ps -ef!!\n";
$match = <MON>;
if ($match) {
print "got fbi match\n" if $DEBUG > 1;
} else {
print "no fbi match\n" if $DEBUG;
# fbi not found
last;
}
close(MON);
print "usleeping, noexist is $noexit\n" if $DEBUG > 1;
usleep($mdelay);
} # end loop testing if fbi has exited
} # close of infinite loop
Optional script
mshowtmp.pl (revision not yet reflected in the tar file)
#!/usr/bin/perl
# add txt_ to beginning of filename
$DEBUG = 1;
$HOME = "/home/pi";
$mshow = "$HOME/mediashow.orig";
$mshow2 = "$HOME/mediashowtmp2";
$ms = `cat $mshow`;
@pics = split('\0',$ms);
$ms = "";
foreach $file (@pics) {
print "file is $file\n" if $DEBUG;
$ms .= "txt_" . $file . "\0";
}
# print out new mediashow pics in order
print "Printing new mediashow: $ms\n" if $DEBUG;
open(MS,">$mshow2") || die "Cannot open mediashow $mshow2!!\n";
print MS $ms;
close(MS);
crontab entries
# display some ip info first
@reboot sleep 15;ip a|grep wlan|sudo tee -a /dev/console > /dev/null
@reboot sleep 22; ./m3.pl >> m3.log 2>&1
# reboot if we can’t reach the Internet
19 5 */2 * * curl google.com > /dev/null 2>&1 || sudo reboot
26 5 */2 * * ./master3.sh >> master.log 2>&1
That will refresh the slideshow every two days, which we found is a good interval for our lifestyle – some days you don’t get around to viewing them. If you want to refresh every day just change ‘*/2″ to ‘*’.
And… that’s it!
Reminder
Don’t forget to make all these files executable. Something like:
$ chmod +x *.pl *.py *.sh
should do it.
My equipment
RPi 3 running Raspbian Lite, OS version “Bullseye,” though older versions also work well, just accommodating the appropriate packages which have changed over time.
Pi Display. The Pi Display resolution is 800×480, so pretty small.
HDMI display such as a TV as alternate to a Pi Display. This does work! I just tested this in Jan, 2022. My Sony TV display resolution is 1920 x 1080.
Pre-install
There are a few things you’ll need (accurate statement as of OS Bullseye, Jan 2022) such as these system packages: fbi, file, rclone, and these python modules: pip, Pillow, and piexif. That’s mostly described in my previous post so I won’t repeat it here. Basically the system package you install with apt-get. After installing pip you use it to install Pillow and piexif.
Getting started
To see how badly things are going for you (hey, I like to be cautiously pessimistic) after you’ve created all these files and have installed rclone, do a
$ ./master3.sh
If you have your rclone file listing (which takes a long time) and want to focusing on debugging the rest of it, do a
$ ./master3.sh skip
Discussion
In this version of Raspberry Pi photo frame I’ve made more effort to force time separation between the randomly selected photos. But, that’s not all. I blow up pictures taken in a narrow (portrait) mode (see next paragraph). And I do some fancy analysis to determine filename, folder, date, time and even location of the pictures. And there’s more. I create an alternate version of each photo which embeds this info at the bottom – in anticipation of my even more fancy remote-controlled slideshow! I am afraid to overwrite what I have previously posted because that by itself is a complete solution and works quite well on its own. So this can be considered worthy of folks looking for a little more challenge to get better results.
The fancyresize.py script is designed around my small PiDisplay which has a horizontal resolution of only 800 pixels. It blows up a narrow, portrait-format picture only if the detected display has a horizontal resolution of no more than 800 pixels. It blows the picture up by 10%, chops off 3% from the top, 7% from the bottom, because that yields optimal results in my experience. If you like that approach but are using a larger HDMI display, you could edit the “801” in that file to make it a larger number (bigger than your display, like 5000).
Show pictures with embedded info
This process is not streamlined. But it can be cool to do it by hand. You could follow these steps.
$ ./mshowtmp.pl; mv mediashowtmp2 mediashow
If you wait the whole cycle the next time around it should display the pictures with the embedded info at the bottom. If you’re impatient, do this:
$ sudo pkill -9 fbi; sudo pkill -9 m3.pl
$ nohup ./m3.pl > m3.log 2>&1 &
googleapi: Error 403: Rate Limit Exceeded, rateLimitExceeded
I’m seeing this while transferring the pictures. Guess I’ll have to slow down the transfer. Not sure. Still figuring this out.
Fun Fact
You know how those old digital cameras created files prefixed with DSC, like DSC00102.JPG? If you read the JPEG spec, which is a pretty dense document, you learn that DSC stands for Digital Still Camera.
Concept for tossing out pictures of documents
We sometimes take pictures of documents, or computer screens, or a slide at a presentation, or a historical marker. They don’t make for compelling slideshow material. Well, the historical markers are debatable since they have character. Anyway, I am looking at using an old open source program called tesseract to do OCR (optical character recognition) on all the photos to help identify those containing a lot of words so they can be excluded. I’ll include that if I determine it to be a good approach.
Installing a searchable dictionary on Raspberry Pi
To install a word dictionary that you can do simple searches against on an RPi, try:
$ sudo apt-get install wamerican
or maybe
$ sudo apt-get install wamerican-huge
Those will produce simple wordlists, not actual dictionaries with definitions as you might have expected. They go into /usr/share/dict, e.g., usr/share/dict/american-english-huge.
The dict program is quite nice. apt-get install dict. Then you run it like this
$ dict neume
and it shoots back definitions and cites sources for those definitions. The drawback for my purposes is that it uses your Internet connection and I’m trying to build a photo frame that doesn’t rely too much on the Internet after the photos themselves are fetched.
$ sudo apt-cache search wordlist
lists all available dictionaries, I believe.
Setting up tesseract
This page has these instructions
git clone https://github.com/thortex/rpi3-tesseract.git
cd rpi3-tesseract
cd release
./install_requires_related2leptonica.sh ./install_requires_related2tesseract.sh
./install_tesseract.sh
But you’re gonna need git first:
$ sudo apt-get install git
RPi lost Wifi
This could be a whole separate post. In the course of my hard work my RPi just would not acquire an IP address on wlan0.
Here’s a great command to see all the SSIDs it knows about:
$ sudo iwlist wlan0 scan > scan.log
Then you can inspect scan.log in an editor. Turns out the one SSID it needed wasn’t in the list. Turns out I had reserved a DHCP entry for it in my router. My router was simply not cooperating, it seems – the RPi wasn’t doing anything wrong. I was almost ready to re-install the whole thing and waste hours… My router is an older model Linksys WRT1200AC. I removed the DHCP reservation on the router, then did a
$ sudo service networking stop; sudo service networking start
on the RPi, and…all was good! Its assigned IP won’t change that often, I can always check the router to see what it is. The management software with the Linksys is quite good.
I’m still having IP issues. So I added an additional crontab entry to display IP info of the WiFi adapter for a few seconds before the slideshow kicks in. This will tell me if it at least has an IP (which it often doesn’t)! It’s a pretty clever idea if I say so myself – the way I managed to do it that is. It’s not in the tar file.
RPi partially blown up
I’ve been running the photo frame for about two years now. All the residents love to see when the pictures refresh what the new slideshow brings. But in all the work I’ve done here and there I’ve partially blown up the RPi. Symptoms: running curl produces a segmentation fault; running crontab -e produces crontab: “/usr/bin/sensible-editor” exited with status 2; and then there’s the fact I lose my IP after a few days. I bet there’s a lot else that’s wrong too, but the sldieshow stuff keeps chugging along, amazingly. I’m too unmotivated (lazy) to fix all these problems, except the IP thing. That prevents slideshow refreshes. So I’ve decided to script a reboot command to run before the slideshow refresh. The resulting conditional reboot is now incorporated into the crontab entries shown earlier.
And by the way, I did fix this problem by re-imaging the micro SD card. That brought a new problem which is that the display blanked out afew only a few seconds. I bought a new display (turns out I didn’t need to), still had the problem, then figured out how to fix it. I wrote up the fix in this post.
Conclusion
A more advanced treatment of photos is shown in this post than I have done previously. It is fairly robust and will withstand quite a few user errors in my experience. The end result will be an interesting display of your photos, randomly selected but in small groupings.
References and related
The tar file which contains everything: https://drjohnstechtalk.com/blog/downloads/photoFrameII.tar
Please see this popular post Raspberry Pi photo frame using your pictures on your Google Drive for more details.
Is your Pi Display blanking out? I have the fix for that in this post.
m3.pl refers to a black.jpg and a newslideshowintro.jpg file. It’s not a disaster to not have those, but the overall experience will be slightly better. Here’s black.jpg:

And the beautiful newslideshowintro.jpg I created is at the top of this blog post.
Tesseract, an surprisingly old and surprisingly good OCR open-source OCR program, is basically impossible to compile for RPi. Fortunately, someone has done it for us. This page has the instructions: https://github.com/thortex/rpi3-tesseract
2 replies on “Raspberry Pi photo frame using the pictures on your Google Drive II”
I am trying this out for my mother-in-law.
It works! Great code! I am studying your code more closely, so I can braid in videos for her too. The plan is that the picture frame rclone connection will be a google account with no method of payment attached, and the albums that people want her to see they share with that id.